question
Devise an algorithm to check if a binary tree is a binary search tree.
A simple check between left and right nodes will not suffice. Think of what happens in
a deeper tree and the criteria numbers at the bottom must fulfill.
A simple check of the left and right child node will not suffice for this. Take a look
at this binary tree:
Because 7 is less than 9, a simple localized check between left and right nodes would not provide the correct answer. Instead we keep track of the minimum and maximum value a node can be at any point in the tree.
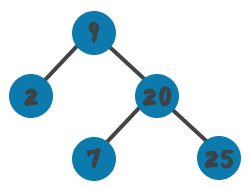
Because 7 is less than 9, a simple localized check between left and right nodes would not provide the correct answer. Instead we keep track of the minimum and maximum value a node can be at any point in the tree.
isBST(Node root)
return isBSTHelper(root, INTEGER_MIN, INTEGER_MAX)
isBSTHelper(Node n, int min, int max)
if (n == null)
return true;
if (n.data > min && n.data < max)
return isBSTHelper(n.left, min, n.data) && isBSTHelper(n.right, n.data, max);
return false;